Meanwhile I changed the script to the following:
Import-Module Adaxes
# Email message settings
$to = "ijacob@littelfuse.com" # TODO: modify me
$subject = "List of the users in lfext.com" # TODO: modify me
$members = Get-AdmUser -Filter 'employeeType -like "extSharePoint"' -Properties * -AdaxesService localhost -Server lfext.com
$htmlBuilder = New-Object "System.Text.StringBuilder"
$htmlBuilder.append("<html><head>")
$htmlBuilder.append("<meta http-equiv=""Content-Type""`
content=""text/html charset=UTF-8""></head>")
$htmlBuilder.append("<body>")
$htmlBuilder.appendFormat(
"<p>Users in lfext.com (<b>{0}</b>)</p>",
$members.count)
$htmlBuilder.append("<table width=""100%%"" border=""1"">")
$htmlBuilder.append("<tr>")
$htmlBuilder.append("<th>User Name</th>
<th>Company</th><th>Title</th><th>Phone</th><th>Mobile</th><th>LF Contact</th><th>LF Title</th><th>LF Mail</th><th>Expiration Date</th><th>Description</th><th>Created</th><th>Modified</th>")
$htmlBuilder.append("</tr>")
foreach ($_ in $members) {
$htmlBuilder.append("<tr>")
$htmlBuilder.appendFormat("<td>{0}</td>", $_.Name)
$htmlBuilder.appendFormat("<td>{0}</td>", $_.company)
$htmlBuilder.appendFormat("<td>{0}</td>", $_.title)
$htmlBuilder.appendFormat("<td>{0}</td>", $_.telephoneNumber)
$htmlBuilder.appendFormat("<td>{0}</td>", $_.mobile)
$htmlBuilder.appendFormat("<td>{0}</td>", $_.mobile) #.mobile is just a placeholder, should be adm-customAttributeText10
$htmlBuilder.appendFormat("<td>{0}</td>", $_.mobile) #.mobile is just a placeholder, should be adm-cumstomAttributeText11
$htmlBuilder.appendFormat("<td>{0}</td>", $_.mobile) #.mobile is just a placeholder, should be adm-cumstomAttributeText12
$htmlBuilder.appendFormat("<td>{0}</td>", $_.accountExpires) # doesn't look like a timestamp
$htmlBuilder.appendFormat("<td>{0}</td>", $_.description)
$htmlBuilder.appendFormat("<td>{0}</td>", $_.createTimeStamp)
$htmlBuilder.appendFormat("<td>{0}</td>", $_.modifyTimeStamp)
$htmlBuilder.append("</tr>")
}
$htmlBuilder.append("</table>")
$htmlBuilder.append("</body></html>")
$Context.SendMail($to, $subject, $NULL,
$htmlBuilder.ToString())
But I'm still facing few issues, phone numbers are not being displayed even so they are definitely filled in, I don't know how to retrieve the adm-CustomAttributeText fields, not sure how to get account expiration date and I'm missing the difference between active, disabled and already expired accounts.
right now, it looks like this:
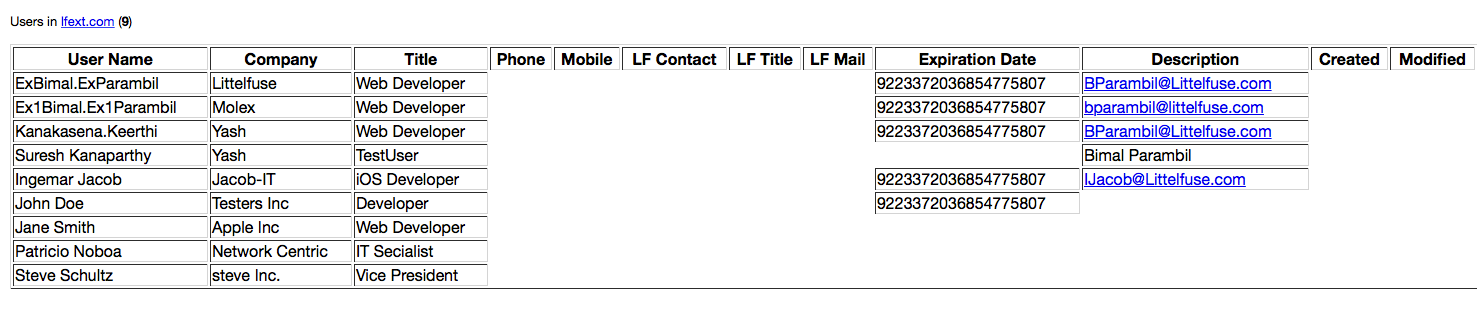