Hello,
Here's a modified version of the script that takes into account your custom UPN suffix (customized to the solution described in userPrincipalName Attribute). In the script, $upnSuffix specifies your custom UPN suffix. Modify it to match your requirements.
Import-Module Adaxes
$upnSuffix = "domain.com" # TODO: modify me
function IsUserNameUnique($username)
{
$user = Get-AdmUser $username -erroraction silentlycontinue
return $user -eq $Null
}
# Get the username
$username = $Context.GetModifiedPropertyValue("samAccountName")
# Check if the username is unique
if (IsUserNameUnique($username))
{
return
}
# If the username is not unique, generate a unique one
$firstName = $Context.GetModifiedPropertyValue("givenName")
$lastName = $Context.GetModifiedPropertyValue("sn")
$uniqueUsername = $Null
if (($firstName -ne $NULL) -and ($lastName -ne $NULL))
{
$username = $lastName
foreach ($char in $firstName.ToCharArray())
{
$username = "$username$char"
if (IsUserNameUnique($username))
{
$uniqueUsername = $username
break
}
}
}
if ($uniqueUsername -eq $NULL)
{
for ($i = 1; $True; $i++)
{
$uniqueUsername = $username + $i;
if (IsUserNameUnique($uniqueUsername))
{
break
}
}
}
# Update User Logon Name (pre-Windows 2000)
$Context.SetModifiedPropertyValue("samAccountName", $uniqueUsername)
# Update User Logon Name
$Context.SetModifiedPropertyValue("userPrincipalName", "$uniqueUsername@$upnSuffix")
$Context.LogMessage("The username has been changed to " + $userLogonName `
+ ".", "Information")
To limit the number of characters in the last name, you'll need to modify the Property Pattern that specifies constraints and value generation templates for the User object type. By default, this is done by the built-in User Pattern. To modify by the built-in User Pattern:
- Launch Adaxes Administration Console.
- Expand the service node that represents your Adaxes service.
- Expand Configuration \ Property Patterns \ Builtin.
- Select the User Pattern. The constraints and value generation templates imposed by this Property pattern will be displayed in the Result Pane (located to the right).
- Click the Add button in the top right corner of the Result Pane.
- Select the Last Name property.
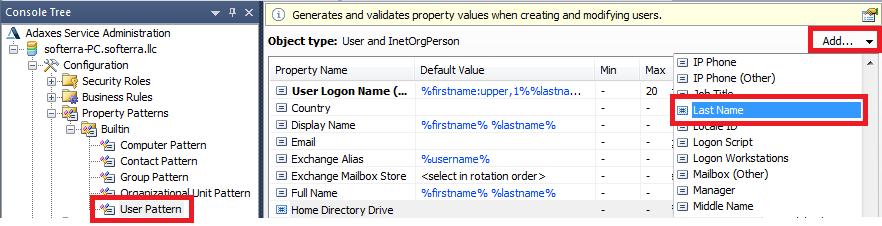
- Check the Maximum length option and type 7 in the associated edit box.
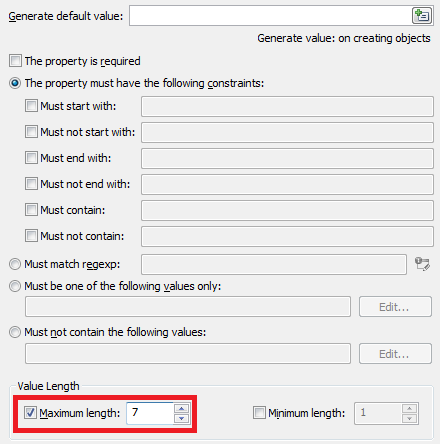
- Click OK and save the Property Pattern.